Here's a solution to convert the given string `S` into a palindrome by replacing question marks with lowercase letters, or returning "NO" if it's not possible:
```python
def solution(S):
S = list(S) # Convert string to list for mutability
N = len(S)
for i in range((N + 1) // 2):
left, right = S[i], S[N - 1 - i]
if left == '?' and right == '?':
S[i] = S[N - 1 - i] = 'a' # Replace both '?' with 'a'
elif left == '?':
S[i] = right # Replace '?' on the left with the right character
elif right == '?':
S[N - 1 - i] = left # Replace '?' on the right with the left character
elif left != right:
return "NO" # If characters do not match and are not '?', it's impossible
return ''.join(S) # Convert list back to string and return
# Examples
print(solution("?ab??a")) # Output: "aabbaa"
print(solution("bab??a")) # Output: "NO"
print(solution("?a?")) # Output: "aaa" or "aza"
```
### Explanation:
1. **Convert the String to a List**: This makes the string mutable, allowing for in-place modifications.
2. **Iterate Over the First Half of the String**: Since a palindrome reads the same forward and backward, you only need to check and modify the first half.
3. **Replace Question Marks**:
- If both characters at positions `i` and `N-1-i` are '?', replace both with 'a'.
- If only one character is '?', replace it with the corresponding character from the other half.
- If both characters are not '?', check if they are equal; if not, return "NO".
4. **Convert Back to String and Return**: After processing, convert the list back to a string.
This approach ensures correctness by handling all possible cases where question marks need to be replaced to form a palindrome. If it detects any impossible condition, it promptly returns "NO".








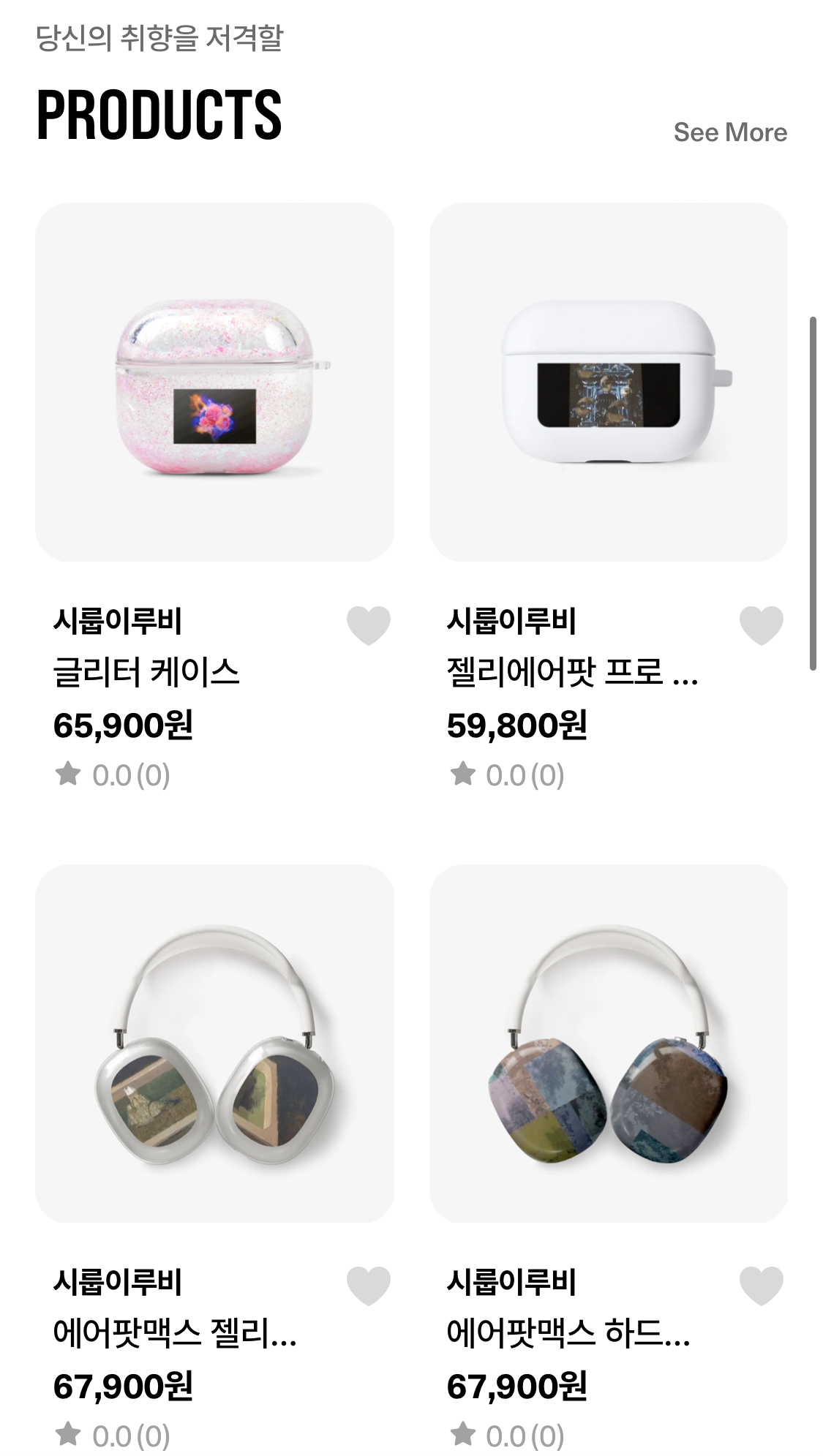

'해외 여행' 카테고리의 다른 글
나는솔로 22기 직업 인스타 갤러리 돌싱특집 영수 광수 순자 포스코 (0) | 2024.09.06 |
---|---|
김뮤즈 블로그 지덕체 컨설팅 55만원 환불 김뮤즈 후기 (0) | 2024.09.06 |
나의 정신건강과 성공 이야기: ADHD, 우울증, 불안증을 극복한 대기업 합격기 (0) | 2024.09.05 |
김뮤즈 y대학교 경제 경영학과 졸업 대기업 여자 부장 변호사 남편 딸 1명 (0) | 2024.09.05 |
김뮤즈 경제 경영학과 졸업 대기업 여자 부장 변호사 남편 딸 1명 (0) | 2024.09.05 |
댓글